
Table of Contents
The Monitor+ Python module gives Python users access to a huge range of reference market data from global exchanges, markets and data vendors. Before you begin, you must have Python version 3 or later installed on your computer and a have valid Monitor+ API key available.
The Python module integrates into Python and makes accessing the market data easy as market data can be accessed with commands beginning with the class name “idata”.
If you do not have already Python version 3 or later (or the Monitor+ Python package) installed
then please click on the install button below.
You will need to have your Monitor+ API key available. If you cannot find the API key,
see the “My Account” section below for help. For any other problems, please see the “Contact Us”
section below.
The “idmmonitor” package has detailed interactive help as can be seen in the Visual Studio Code and PyScripter example screenshots below. Type “idata.” in the console window on the left and a list of the available commands is displayed. Many of the help topics also have clickable links to open the relevant section in the online Monitor+ API help system.
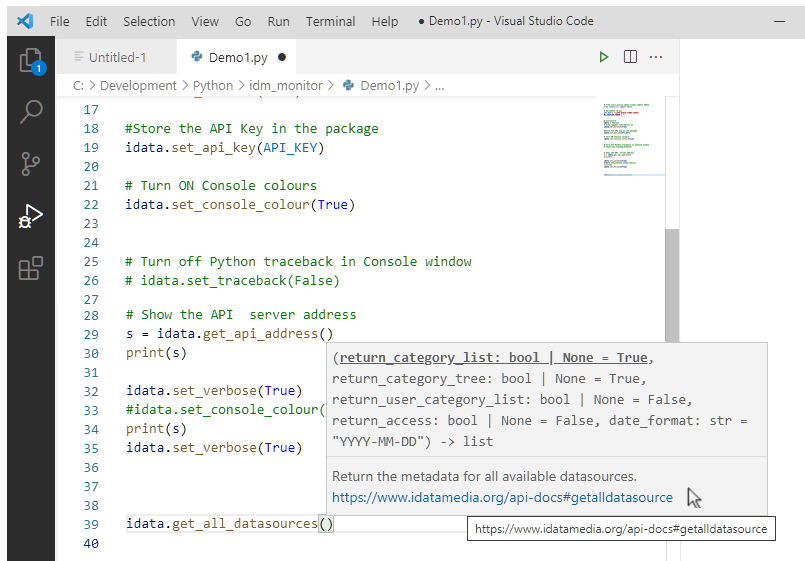
The Python module is a subset of the full Monitor+ API. You can view the API documentation by clicking on the “Documents” menu at the top of this window and selecting the “API Documentation” menu (see the image below).
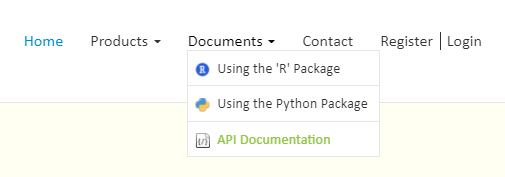
The API documentation shows in detail the available functions and their parameters and should be referenced when writing Python code that uses the Monitor+ Python module. The API, “R” and Python function and parameter names are very similar to make the online documentation familiar to all developers. Note that not all API functions or parameters are available (or needed) in the Python module.
If you need further assistance please go to the “Contact Us” section below.
You will need your Monitor+ API key to download the Python module. You should have received your API key from us via email. You can also access your API key by logging in using the top right "Login" menu at the top of this page, logging in and viewing your account. If you are already logged in, your user name will be displayed on this menu (see the red box in the image below).
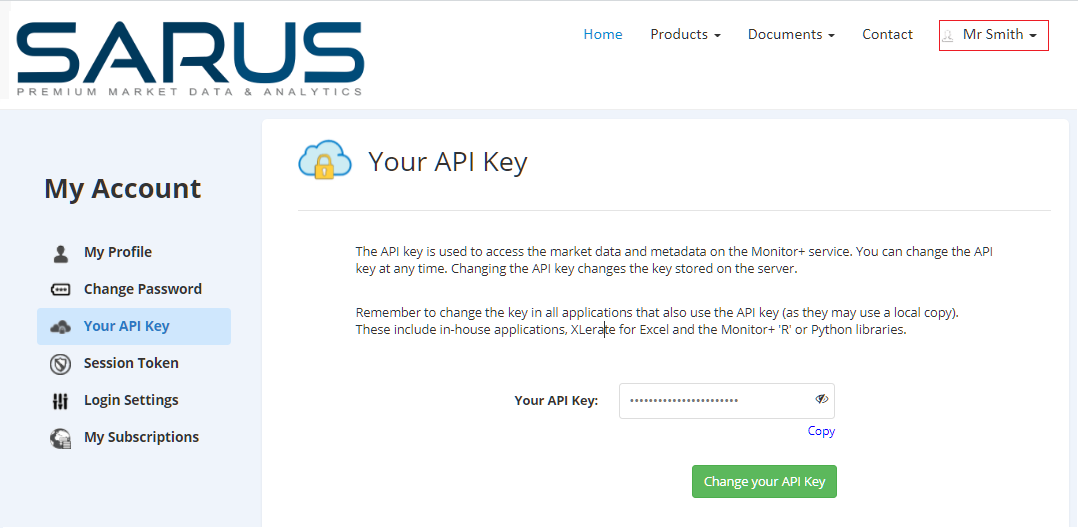
Click on the symbol in the edit box to see the API key. Click the “Copy” link to copy the API key to the clipboard.
If you still have a problem locating your API key, please see the "Contact us" section below.
You will need your Monitor+ API key to access the data on the service. If you have a problem locating your API key, please see the "Locating your API key" section above.
To begin you need to initilise the ‘idmmonitor’ module, store your API key and get a session token from the remote API server. You need to type four commands (in black text) below in the Python console to get a session token. In the example below, commands are in black text, comments in green and results from the server in blue.
# from class_source.idata_client import IData
>>> from idmmonitor import IData
# Initialise the class
>>> idata = IData()
# Store your API key in the module
> >> idata.set_api_key("XXXXX-XXXXX-XXXXX-XXXXX")
Stored the new API key XXXXX-XXXXX-XXXXX-XXXXX.
# Get a session token
[INFO] Retrieving session token using: XXXXX-XXXXX-XXXXX-XXXXX
[INFO] API call to: http://api.sarus.com/GetSessionToken?APIKey=
XXXXX-XXXXX-XXXXX-XXXXX
[INFO] Session token retrieved: 12ba9c13a8c9f270e71bb2526f0532ad
Once you have a valid session token, you can access the full service. As you can see above, commands to the Monitor+ package are prefixed with the term “idata.”.
A full list of functions available in the package is located at the bottom of this document as well as Python coding examples.
Session tokens are needed to access data and metadata. Session tokens expire after a number of minutes of inactivity (from 10-30 minutes depending on server settings). The Monitor+ package will automatically attempt to get a new session token if the current one has expired. You can check the life remaining in the current session token with the query_session_token command below. You can also use this command to test that communications with the API server is OK.
After you receive a session token, you should be able to list datasources and their datasets and download values for any datasets that you have access to. You can check what datasources you can access using the idata.get_user_datasources command.
The Monitor+ API allows you to build, structure and manage a list of user “favorite” or frequently used datasets (time series). This ‘favorites’ list is designed to allow the user to create and manage their own selection of datasets from the many tens of thousands that may be available with their subscription. The favorites list is also is very useful for creating applications for end users.
There are 4 user "favorite" commands in the "idata" class to help manage your favorite datasets. They are get_user_favorite_status(), get_user_favorites(), add_user_favorites() and remove_user_favorites().
Please look at the available Python functions table at the end of this document for more details. Also look at the API documentation for more detailed information on using each function. See the "The Monitor+ API documentation" section above for details.
You can also manage your list of favourite datasets using your user account menu and selecting the "My Favorites" menu. After logging in, the “Register/Login” top menu will display your user name (See the red box in the image below). Click on this menu to access the “My Favorites” page.
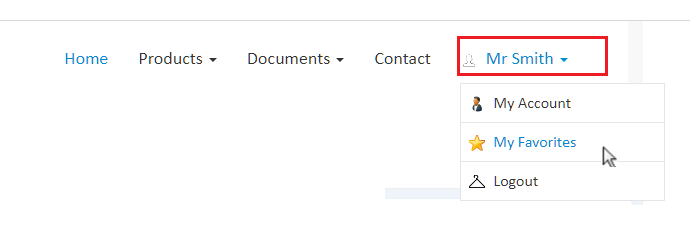
You can view your account details online by logging in using the "Login" menu above at the top right of this page. If you are already logged in, your user name will be displayed on this menu (see the image above).
To login you will need your User Reference Number, user name and password (these should have been emailed to you). Click on the "Login" menu and enter your reference number, user name and password. Once logged in, click on the the top right menu (with your user name) to access your account. From here you can see your datasources, reset your password or API key, manage your user favorites and more.
If you have problems accessing your account page, please check your emails page, contact your IT colleagues or see the “Contact us” section below.
For general enquiries please click the “Contact” menu at the top of the window above and select the contact choice you require.

For technical support you can also contact us via email. Please email support@sarus.com with a subject of “Monitor+ Python Module question”. Send your contact details, User Reference Number and description of the issue and any images, output or screenshots that may assist.
Please note we do not offer advice with any Python setup or programming issues, only issues regarding accessing data from the API. Also, please see the Python examples section below for more examples on using the Python module.
Below are examples of using the “IData.” Python module. In the example 4, you can see how to access the objects returned from the API. In the examples below, commands are in black text, comments in green and the server results in blue.
# Example 1. Echo request information on
>>> idata.set_verbose(True)
[INFO] Verbose settting updated to True
# Exampe 2. Turn off traceback in Console window
>>> idata.set_traceback(False)
[INFO] Traceback settting updated to False
# Example 3. Add two contracts to your user favorites list
idata.add_user_favorites (["ECBFX/EURGBP",
"ECBFX/EURCAD"])
{'Added': 2, 'Ignored': 0, 'Status': 200}
# Print user datasource information. No result from server is displayed due to space limitations.
# No error checks are made
result = idata.get_user_datasources(return_category_list=True, return_category_tree=True)
for i in result: print("Keys", list(i.keys())) print("Datasource: ", i['Datasource']) print("Name: ", i['Name']) print("HasCategoryTree: ", i['HasCategoryTree']) print('Details:') print("SeriesCount: ", i['Details']['SeriesCount']) print("StartDate: ", i['Details']['StartDate']) print("EndDate: ", i['Details']['EndDate']) if i['HasCategoryTree']: print("Category Tree", i["Details"]["CategoryTree"])
Function | Description |
---|---|
set_api_key | Store your API key in the Python module. |
print_api_key() | Return the API key that was set with the set_api_key command. |
get_api_address() | Displays the selected API server IP address. |
get_api_version() | Returns the API version number from the API server. |
request_new_api_key() | Return a new API key. |
get_session_token() | Return a new session token. |
query_session_token() | Return the remaining token life in milliseconds. |
renew_session_token() | Renew the current token to full life. |
revoke_session_token() | Invalidate the current token on the server. |
print_session_token() | Return the current session token value. |
get_datasource() | Return the metadata for one named datasource. |
get_all_datasources() | Return the metadata for all available datasources. |
get_user_datasources() | Return the metadata datasources user can access. |
get_datasets() | Return metadata for the datasets in one datasource |
get_selected_datasets() | Return metadata for multiple named datasets in one or more datasources. |
get_dataset_values() | Return a range dataset values or averages for named datasets in one or more datasources. |
get_dataset_values_rc() | As get_dataset_values but values returned in row x column format. |
get_dataset_values_for_date() | Return dataset values or averages for named datasets (in one or more datasources) for a single date. |
get_user_favorite_status() | Return the server date and time that the user favorites list was last changed. |
get_user_favorites() | Return metadata for all datasets in your user favorites list. |
add_user_favorites() | Add named datasets to your user favorites list. |
remove_user_favorites() | Remove named datasets from your user favorites list. |
get_my_account_details() | Return registered user account details including API key. |
set_return_raw_data(true) | If true return raw JSON from all server requests. |
set_verbose(true) | If true extra the request details are displayed in the console. |
set_traceback(True) | Turn on/off traceback in the Python console window. |